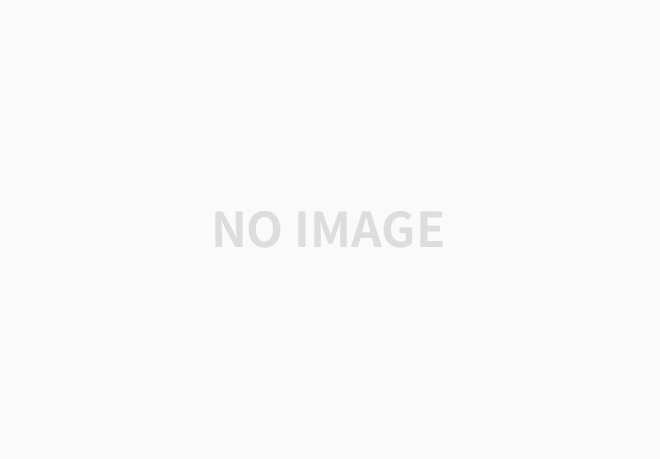
1. Overloading
→ 실제로 많은 오버로딩된 함수를 직접 작성하지는 않을 것이다
→ 하지만, 다른 사람들이 만든 외부 라이브러리를 사용할텐데 이런 패키지나 라이브러리들은 오버로딩을 많이 사용한다
→ 함수가 서로 다른 여러개의 Call Signature를 가지고 있을때 발생시킴
type Add = {
(a:number, b:number) : number,
(a:number, b:string) : number
}
const add:Add = (a, b) => {
if(typeof b ==="string") return a
return a + b
}
// 이렇게 작성할 수 있지만 별로 좋지 못한 코드
type Config = {
path: string,
state: object
}
type Push = {
(path: string): void,
(config: Config): void
}
const push: Push = (config) => {
if(typeof config === "string") { console.log(config) }
else { console.log(config.path) }
}
1.1. Call Signiture의 파라미터 개수가 다른 경우
type Add = {
(a:number, b:number): number,
(a:number, b:number, c:number): number
}
const add:Add = (a, b, c) => {
return a + b
} // 이렇게 작성하면 TS는 오류를 반환한다.
const add:Add = (a, b, c?:number) => {
if(c) return a + b + c // c가 있을 경우, a + b + c return
return a + b // c가 없을 경우, a + b return
} // 이렇게 c라는 파라미터는 옵션임을 알려줘야 한다.
add(1, 2)
add(1, 2, 3)
// 둘 다 잘 작동하게 된다
이 경우는 파라미터의 개수가 다를 때 일어나는 경우이다
그 의미는 마지막에 적힌 c라는 추가 파라미터가 옵션이라는 뜻이다
따라서 추가적으로 타입을 줘야하고, 이 파라미터는 선택사항이라는 것을 알려줘야 한다
2. Polymorphism (다형성)
- Many(poly) + Structure(morphos) → 여러가지 다른 구조물
- 다형성은 다른 모양의 코드를 가질 수 있게 해주는 것
- 여러가지 다른 모양들, 여러가지 다른 형태들
예시) 배열을 만들어 입력을 받고 배열 속 요소의 타입에 상관없이 하나씩 출력하는 함수
type SuperPrint = {
(arr: number[]): void
(arr: boolean[]): void
// (arr: string[]): void
}
const superPrint: SuperPrint = (arr) => {
arr.forEach(i => console.log(i))
}
superPrint([1, 2, 3, 4])
superPrint([true, false, true, true])
superPrint(["a", "b", "c"]) // 이 부분에서 오류가 발생할 것이다.
// string 타입에 대한 부분을 작성하지 않았기 때문이다.
// 이것을 해결하기 위해 string 타입을 작성하는 것 보다 다형성을 사용하는 것이 좋다.
superPrint([1, 2, true, false]) // 이 부분도 오류가 발생할 것이다.
// superPrint 함수의 목적은 안에 요소의 타입이 어떻든 출력하는 것이다.
2. 2
'개발 > TypeScript' 카테고리의 다른 글
9. 타입스크립트(TypeScript) - Class (0) | 2022.09.22 |
---|---|
8. 타입스크립트(TypeScript) - Concrete Type & Generic Type (0) | 2022.09.04 |
6. 타입스크립트(TypeScript) - 타입 & Call Signature (0) | 2022.09.04 |
5. 타입스크립트(TypeScript) - Readonly & Tuple (0) | 2022.09.04 |
4. 타입스크립트(TypeScript) - Alias(별칭) & return 타입 지정 (0) | 2022.09.03 |